
|
Downloads
Demo: geexlab-demopack-gl21/d65-curl/main.xml
How to run the demo?
Download and unzip GeeXLab and the demopack where you want, launch GeeXLab and drop the demo (main.xml) in GeeXLab. This demo requires OpenGL 2.1+. |
curl is a powerful command line utility for transferring data with URLs. curl has a lot of command line parameters that offer a myriad of possibilities.
For example, if you want to download the following image (in this tweet) with curl from the command line, just type:
curl --insecure -o img.jpg https://pbs.twimg.com/media/FVXVWBEXEAAvr69?format=jpg
and you should get the following image (file img.jpg) in the folder:

We can do the same thing with GeeXLab in Lua or Python. Here are the current functions to deal with curl engine available in the gh_utils lib:
– curl_get_file_time_and_size()
– curl_download_file_in_buffer()
– curl_download_file_v1()
curl_get_file_time_and_size() allows to get the time and size of a remote file. Useful to check the presence of a file before trying to download it.
curl_download_file_v1() downloads a remote file and store it in a regular file on the disk. In the case of an image for example, you can then load the texture with gh_texture.create_from_file().
curl_download_file_in_buffer() downloads a remote file and store it in a memory buffer. Still in the case of an image, you can create a texture from the buffer with gh_texture.create_from_buffer().
curl functions are available on Windows, Linux and Raspberry Pi OS. GeeXLab for Windows comes with curl DLLs. On Linux and Raspberry Pi OS, curl is already installed and you have to tell GeeXLab where to find the .so files.
The demo (d65-curl/) shows how to use these functions:
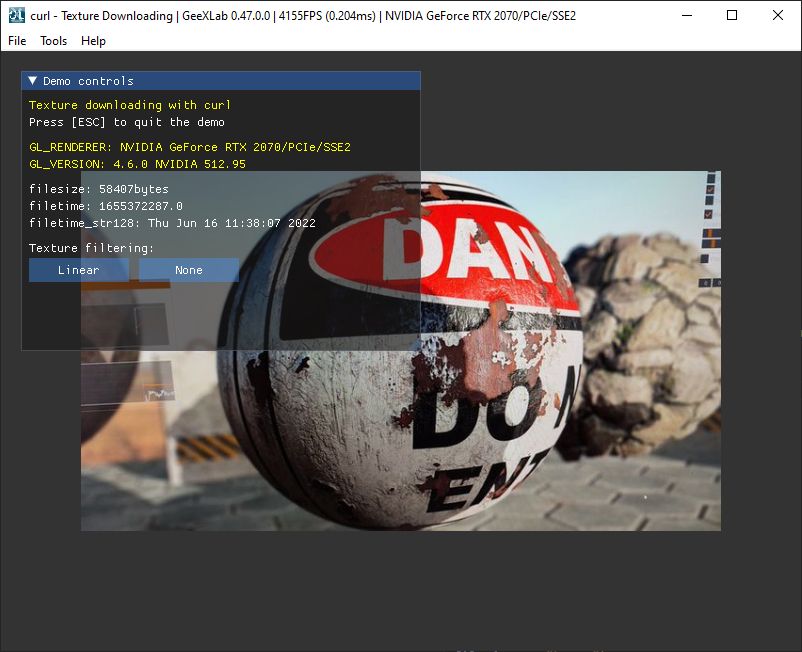
Here is a code snippet with the important functions (complete code is available in init.lua):
local remote_filename = "https://pbs.twimg.com/media/FVXVWBEXEAAvr69?format=jpg&name=small"
local app_dir = gh_utils.get_app_dir()
curl_dylib = "" -- On Windows
if (is_linux) then
curl_dylib = app_dir .. "dylibs/libcurl.so.4.6.0" -- Linux Mint 20.3
elseif (is_rpi) then
curl_dylib = app_dir .. "dylibs/libcurl.so.4.7.0" -- Raspberry Pi OS
end
-- Check the size of the file that will be downloaded. This step is optional, you can directly download the file from the web.
--
filesize, filetime, filetime_str128 = gh_utils.curl_get_file_time_and_size(curl_dylib, remote_filename)
if (filesize > 0) then
local download_in_memory = true
if (download_in_memory) then
-- Download the file in a memory buffer without storing it in a file on the disk.
--
buffer, buffer_size = gh_utils.curl_download_file_in_buffer(curl_dylib, remote_filename)
if (buffer_size > 0) then
tex0 = gh_texture.create_from_buffer(buffer, buffer_size, upload_to_gpu, pixel_format, texture_unit, gen_mipmaps, compressed_texture)
gh_utils.curl_free_buffer(buffer)
end
else
-- Download the file in a local file on the disk.
--
local local_filename = demo_dir .. "assets/01.jpg"
local ret = gh_utils.curl_download_file_v1(curl_dylib, remote_filename, local_filename)
if (ret == 1) then
if (file_exists(local_filename)) then
tex0 = gh_texture.create_from_file_v6(local_filename, pixel_format, gen_mipmaps, compressed_texture)
end
end
end
end